- Daily Dose of Data Science
- Posts
- The Most Common Misconception About __init__() Method in Python
The Most Common Misconception About __init__() Method in Python
Demystifying the __init__() method.
Most Python programmers misinterpret the __init__() magic method in Python OOP. They think that it creates a new object, i.e., allocates memory to it.
For instance, consider the Point2D class below:

When we create an object (shown below), programmers believe that in the background, it is the __init__() method that is allocating memory to their object:

But that is not true.
When we create ANY object in Python, the __init__() method NEVER allocates memory to it.
As the name suggests, __init__() only assigns value to an object’s attributes, i.e., initialize the attributes.
Instead, it’s the __new__() magic method that creates a new object and allocates memory to it.
To understand better, consider the class implementation below.

Here, we have implemented the __new__() method, which checks if the passed arguments are of integer type.
Now, if we try to create an object of this class, Python would first validate the checks specified in the __new__ () method and create a new object only when the specified conditions are true.
This is evident from the image below:
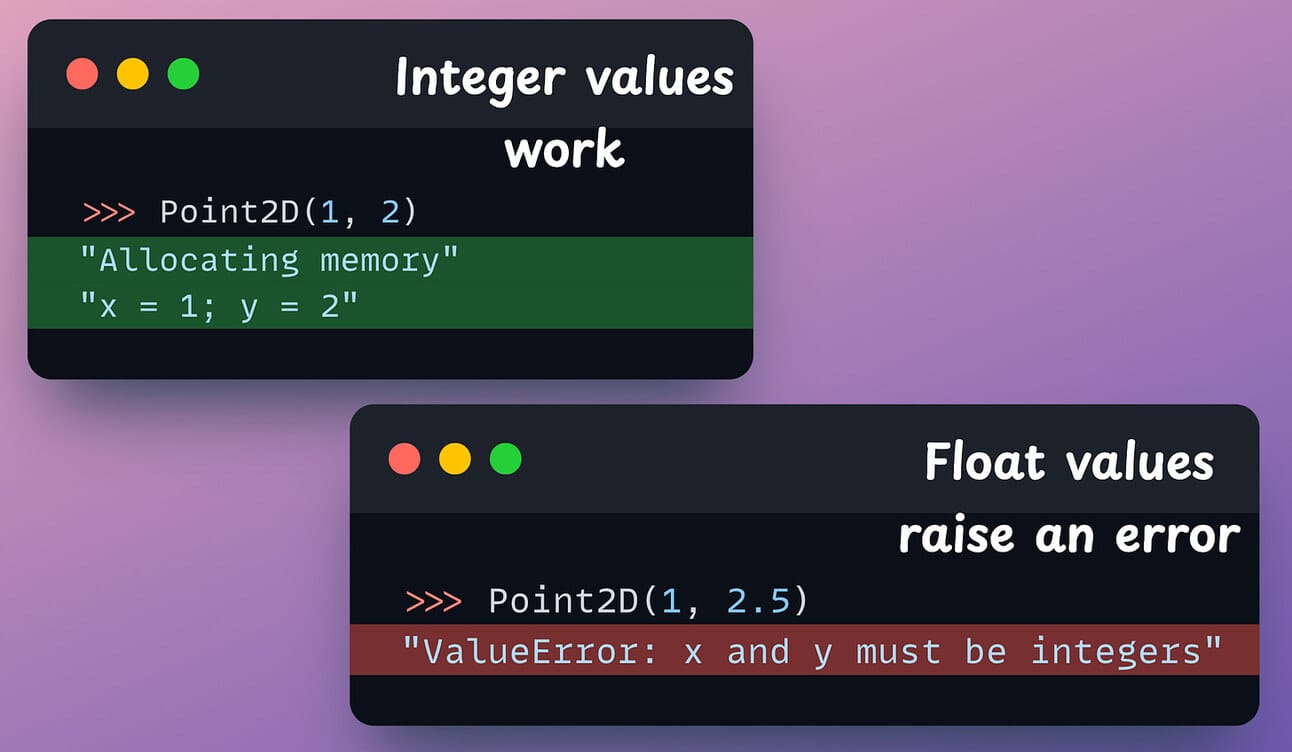
Another popular use case of the __new__ method is to define singleton classes — classes that can only have one object.
For instance, consider the following class implementation:

In the above code, the __new__ method define a class variable _class_count.
When a new object is instantiated, if the value of _class_count=0, the value of _class_count is updated to 1 and the object is returned.
After creating the first object, a new object can never be created because the value of _class_count will never be 0.
This is evident from the image below:

Here’s a full deep dive into Python OOP if you want to learn more about advanced OOP in Python: Object-Oriented Programming with Python for Data Scientists.
👉 Over to you: What are some other use cases of __new__ magic method?
👉 If you liked this post, don’t forget to leave a like ❤️. It helps more people discover this newsletter on Substack and tells me that you appreciate reading these daily insights.

The button is located towards the bottom of this email.
Thanks for reading!
Latest full articles
If you’re not a full subscriber, here’s what you missed last month:
Don’t Stop at Pandas and Sklearn! Get Started with Spark DataFrames and Big Data ML using PySpark.
DBSCAN++: The Faster and Scalable Alternative to DBSCAN Clustering
Federated Learning: A Critical Step Towards Privacy-Preserving Machine Learning
You Cannot Build Large Data Projects Until You Learn Data Version Control!
Deploy, Version Control, and Manage ML Models Right From Your Jupyter Notebook with Modelbit
To receive all full articles and support the Daily Dose of Data Science, consider subscribing:
👉 Tell the world what makes this newsletter special for you by leaving a review here :)
👉 If you love reading this newsletter, feel free to share it with friends!
Reply