- Daily Dose of Data Science
- Posts
- The Most Misunderstood Thing About a Tuple's Immutability
The Most Misunderstood Thing About a Tuple's Immutability
...and here's what immutability actually means.
When we say tuples are immutable, most Python programmers think that the values inside a tuple cannot change.
But this is not entirely true.
For instance, in the code below, we have a tuple whose second element is a list.
Appending to the list updates the tuple’s value, and yet, Python does not raise an error:

What happened there?
If tuples were really immutable, then Python must have raised an error on the append statement, no?
But as demonstrated above, it didn’t.
So it’s certain that we are missing something here.

This brings us to an important point that I have seen many tutorials/courses overlooking.
When we say tuples are immutable, it means that during its entire lifecycle, two things can NEVER change:
The object IDs inside a tuple.
The order of object IDs inside a tuple.
For instance, say a tuple has two objects with object IDs — a and b.

Immutability says that this tuple will always continue to reference only two objects:
With IDs “a” and “b”.
And their IDs will be in the order: “a” followed by “b”.

But there is NO restriction that these individual objects cannot be modified.
Thus, even if the objects inside a tuple are mutable, we can safely modify them, and this will not violate a tuple’s immutability.
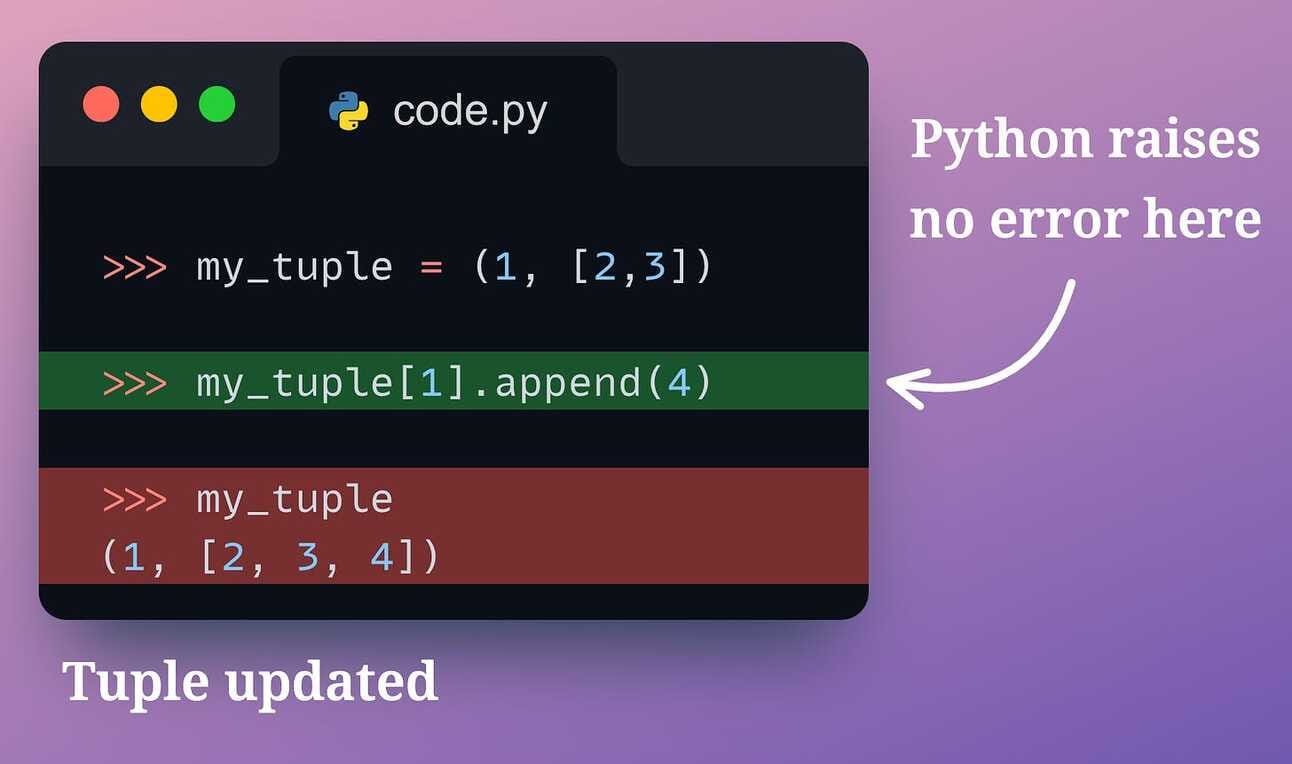
This explains the demonstration above.
As append performs an inplace operation, the collection of IDs inside the tuple never changed.
Thus, Python didn’t raise an error.
In fact, we can also verify this by printing the collection of object IDs referenced inside the tuple before and after the append operation:

As shown above, the object IDs before the append operation and after the append operation are the same.
Thus, immutability isn’t violated and Python never raised an error.
Cool stuff, isn’t it?
👉 Over to you: What are some other overlooked aspects of Python programming?
👉 If you liked this post, don’t forget to leave a like ❤️. It helps more people discover this newsletter on Substack and tells me that you appreciate reading these daily insights.

The button is located towards the bottom of this email.
Thanks for reading!
Latest full articles
If you’re not a full subscriber, here’s what you missed last month:
You Cannot Build Large Data Projects Until You Learn Data Version Control!
Why Bagging is So Ridiculously Effective At Variance Reduction?
Sklearn Models are Not Deployment Friendly! Supercharge Them With Tensor Computations.
Deploy, Version Control, and Manage ML Models Right From Your Jupyter Notebook with Modelbit
Model Compression: A Critical Step Towards Efficient Machine Learning.
Gaussian Mixture Models (GMMs): The Flexible Twin of KMeans.
To receive all full articles and support the Daily Dose of Data Science, consider subscribing:
👉 Tell the world what makes this newsletter special for you by leaving a review here :)
👉 If you love reading this newsletter, feel free to share it with friends!
Reply