- Daily Dose of Data Science
- Posts
- 10 Ways to Declare Type Hints in Python
10 Ways to Declare Type Hints in Python
Must-know for Python programmers.
When writing Python code, type hints provide an incredible way to specify explicit information about:
the expected types of function arguments, and
their return type.
Yet, we all know that Python NEVER enforces them.

But does that mean they have no utility?
Of course not!
Type hints are becoming increasingly valuable to Python projects lately.
Despite not being enforced, Python developers find them extremely useful to improve code quality and maintainability.
So today, let me walk you through 10 different and must-know ways to declare type hints in just two minutes.
Let’s begin!
#1) Type hints for standard Python objects
The most basic (and must-know) way to declare type hints for standard Python objects is as follows:

Everyone knows the above type hints as these are quite common.
For list, tuple and dict specifically, however, it is recommended to use the typing module instead because it lets us provide more information about the object:

#2) Multiple type hints for an object
If an object can have multiple types, use the Union keyword (or the pipe symbol if you are using Python 3.10 or above):

#3) Types hints for None values
Sometimes, an object can be None or have any other standard data type(s). Declare such objects as follows:

#4) Type hints for iterable
Iterables are objects you can iterate on — list, tuple, or dict.
Declare such objects as follows:

#5) Type hints for constant objects
Some objects can never change their value throughout the program. They must remain constant. Declare them using Final as follows:

#6) Type hints for fixed values
Some objects may not be constant but may only take a fixed set of values. For instance, grade may only take values from a fixed set of grades.
Declare such variables as follows:
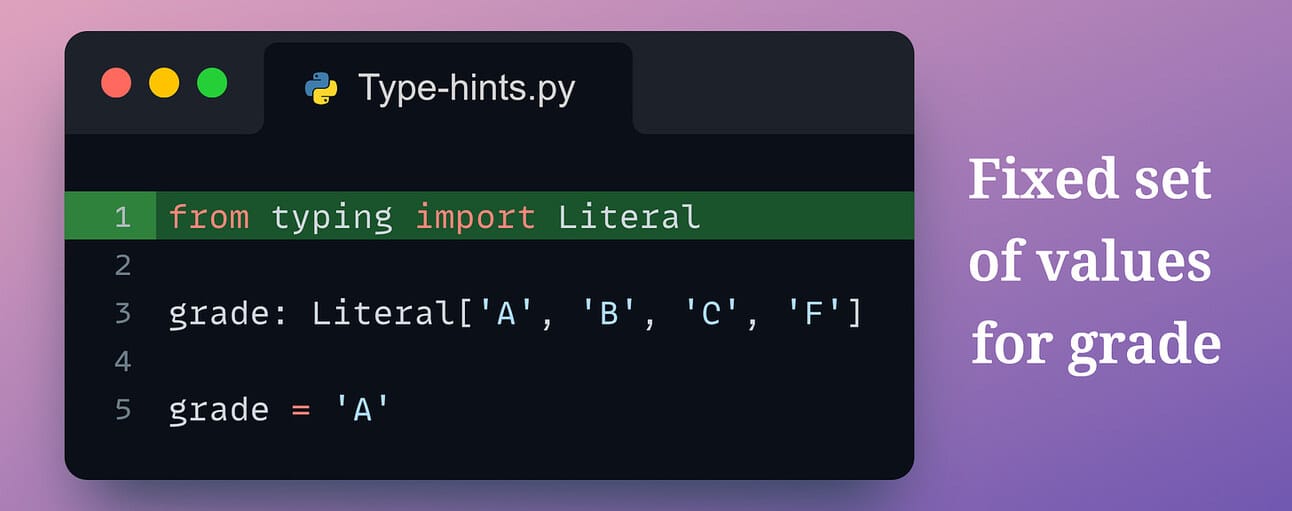
#7) Type hints for objects with any possible value
Some objects could be of any type — list, int, string, etc. Declare them as follows:

#8) Type hints in functions
We declare type hints in functions in two places:
To specify type hints for its parameters.
To specify the type hint for the object returned by the function.

You can use any of the above discussed type hints to declare type hints in functions — Any, Union, Iterable, Optional etc.
#9) Type hint of a function object
At times, a function may have another function as its parameters. This happens all the time in Python decorators.
We can declare the data type for such parameters as follows:

Callable, as the name suggests, is any object that can be invoked — object().
#10) Type hint aliasing
If type hints become big, complex, and unreadable, we can alias them by declaring shorter names for them:

Done!
These are the 10 most common and must-know ways to declare type hints in Python.
Of course, as discussed earlier, Python never enforces type hints.
Yet, they are immensely valuable and a must-know skill if you want to write better-documented, more reliable, and maintainable code.
I created this Jupyter Notebook for you to get started with Type Hints: Type hints notebook.
👉 Over to you: What type hints did I miss?
Whenever you are ready, here’s one more way I can help you:
Every week, I publish 1-2 in-depth deep dives (typically 20+ mins long). Here are some of the latest ones that you will surely like:
[FREE] A Beginner-friendly and Comprehensive Deep Dive on Vector Databases.
A Detailed and Beginner-Friendly Introduction to PyTorch Lightning: The Supercharged PyTorch
You Are Probably Building Inconsistent Classification Models Without Even Realizing
Why Sklearn’s Logistic Regression Has no Learning Rate Hyperparameter?
PyTorch Models Are Not Deployment-Friendly! Supercharge Them With TorchScript.
Federated Learning: A Critical Step Towards Privacy-Preserving Machine Learning.
You Cannot Build Large Data Projects Until You Learn Data Version Control!
To receive all full articles and support the Daily Dose of Data Science, consider subscribing:
👉 If you love reading this newsletter, feel free to share it with friends!
👉 Tell the world what makes this newsletter special for you by leaving a review here :)
Reply